osi3::TrafficLight::Classification Struct Reference
Classification
data for a traffic light.
More...
Collaboration diagram for osi3::TrafficLight::Classification:
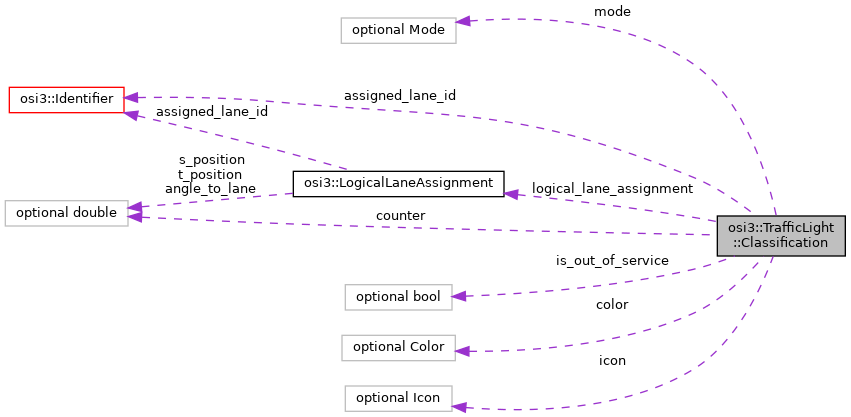
Public Types
Public Attributes
optional Color | color = 1 |
The semantic color of the traffic light. More... | |
optional Icon | icon = 2 |
The icon of the traffic light. More... | |
optional Mode | mode = 3 |
The operating mode of the traffic light. More... | |
optional double | counter = 4 |
The value of the countdown counter. More... | |
repeated Identifier | assigned_lane_id = 5 |
The IDs of the lanes that the traffic light is assigned to. More... | |
optional bool | is_out_of_service = 6 |
Boolean flag to indicate that the traffic light is taken out of service. More... | |
repeated LogicalLaneAssignment | logical_lane_assignment = 7 |
Assignment of this object to logical lanes. More... | |
Detailed Description
Classification
data for a traffic light.
Member Enumeration Documentation
◆ Color
Definition of semantic colors for traffic lights.
- Note
- The color types represent the semantic classification of a traffic light only. They do not represent an actual visual appearance.
◆ Icon
Definition of traffic light bulb icon.
◆ Mode
Definition of light modes for traffic lights.
Member Data Documentation
◆ color
optional Color osi3::TrafficLight::Classification::color = 1 |
The semantic color of the traffic light.
- Note
- The color types represent the semantic color classification of a traffic light only. They do not represent an actual visual appearance.
-
If the color of the traffic light is known (from history or geometrical arrangement) and the state
mode
isMODE_OFF
thencolor
could remain unchanged. If traffic light displays images in different colors and traffic light is off (mode
=MODE_OFF
), thencolor
=COLOR_OTHER
.
◆ icon
optional Icon osi3::TrafficLight::Classification::icon = 2 |
The icon of the traffic light.
◆ mode
optional Mode osi3::TrafficLight::Classification::mode = 3 |
The operating mode of the traffic light.
◆ counter
optional double osi3::TrafficLight::Classification::counter = 4 |
The value of the countdown counter.
Unit: % or s
- Note
- Set value only if traffic light bulb is a countdown counter.
- Rules
- is_greater_than_or_equal_to: 0
◆ assigned_lane_id
repeated Identifier osi3::TrafficLight::Classification::assigned_lane_id = 5 |
The IDs of the lanes that the traffic light is assigned to.
Might be multiple if the traffic light is valid for multiple driving lanes.
- Note
- OSI uses singular instead of plural for repeated field names.
- Rules
- refers_to: Lane
◆ is_out_of_service
optional bool osi3::TrafficLight::Classification::is_out_of_service = 6 |
Boolean flag to indicate that the traffic light is taken out of service.
This can be achieved by visibly crossing the light, covering it completely or switching the traffic light off.
◆ logical_lane_assignment
repeated LogicalLaneAssignment osi3::TrafficLight::Classification::logical_lane_assignment = 7 |
Assignment of this object to logical lanes.
- Note
- OSI uses singular instead of plural for repeated field names.
- osi_trafficlight.proto